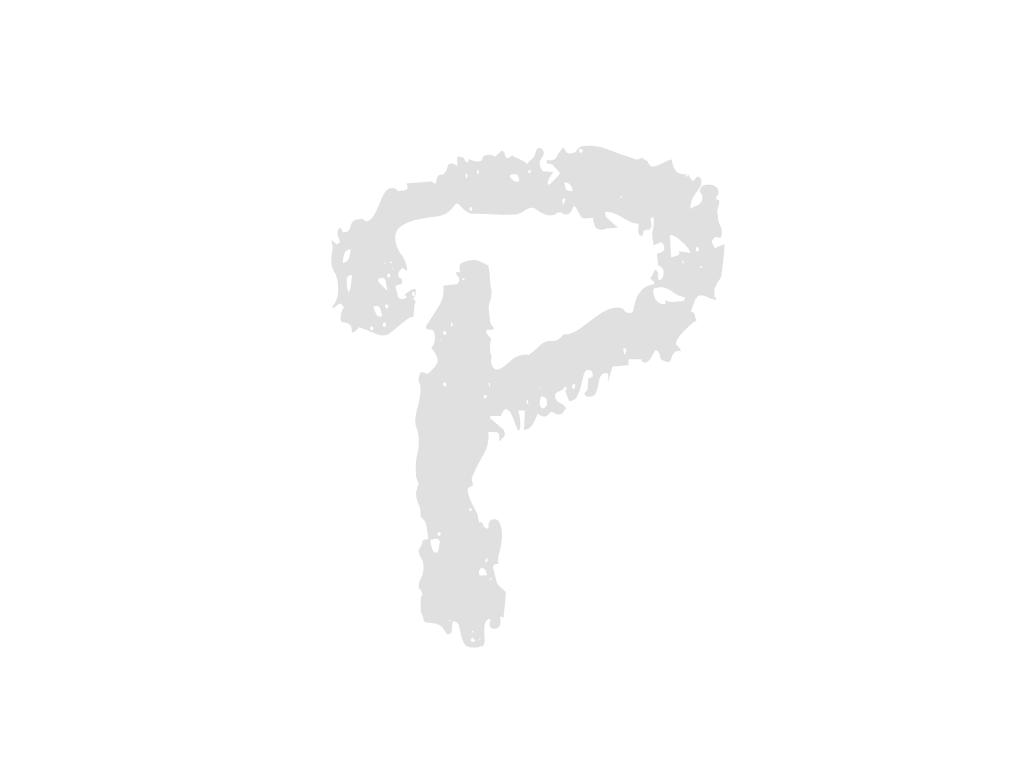
--- 202108/.classpath
+++ 202108/.classpath
... | ... | @@ -1,13 +1,14 @@ |
1 | 1 |
<?xml version="1.0" encoding="UTF-8"?> |
2 | 2 |
<classpath> |
3 |
- <classpathentry kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8"/> |
|
3 |
+ <classpathentry exported="true" kind="con" path="org.eclipse.jdt.launching.JRE_CONTAINER/org.eclipse.jdt.internal.debug.ui.launcher.StandardVMType/JavaSE-1.8"/> |
|
4 | 4 |
<classpathentry kind="src" path="src/main/java"/> |
5 |
- <classpathentry kind="con" path="org.eclipse.jst.server.core.container/org.eclipse.jst.server.tomcat.runtimeTarget/Apache Tomcat v9.0"> |
|
5 |
+ <classpathentry exported="true" kind="con" path="org.eclipse.jst.server.core.container/org.eclipse.jst.server.tomcat.runtimeTarget/Apache Tomcat v9.0"> |
|
6 | 6 |
<attributes> |
7 | 7 |
<attribute name="owner.project.facets" value="jst.web"/> |
8 | 8 |
</attributes> |
9 | 9 |
</classpathentry> |
10 | 10 |
<classpathentry kind="con" path="org.eclipse.jst.j2ee.internal.web.container"/> |
11 | 11 |
<classpathentry kind="con" path="org.eclipse.jst.j2ee.internal.module.container"/> |
12 |
+ <classpathentry exported="true" kind="lib" path="C:/project/mariadb-java-client-2.7.3.jar"/> |
|
12 | 13 |
<classpathentry kind="output" path="build/classes"/> |
13 | 14 |
</classpath> |
+++ 202108/src/main/java/Action.java
... | ... | @@ -0,0 +1,49 @@ |
1 | + | |
2 | + | |
3 | +import java.io.IOException; | |
4 | +import java.io.PrintWriter; | |
5 | + | |
6 | +import javax.servlet.ServletException; | |
7 | +import javax.servlet.annotation.WebServlet; | |
8 | +import javax.servlet.http.HttpServlet; | |
9 | +import javax.servlet.http.HttpServletRequest; | |
10 | +import javax.servlet.http.HttpServletResponse; | |
11 | + | |
12 | +/** | |
13 | + * Servlet implementation class Action | |
14 | + */ | |
15 | +@WebServlet("/Action") | |
16 | +public class Action extends HttpServlet { | |
17 | + private static final long serialVersionUID = 1L; | |
18 | + | |
19 | + /** | |
20 | + * @see HttpServlet#HttpServlet() | |
21 | + */ | |
22 | + public Action() { | |
23 | + super(); | |
24 | + // TODO Auto-generated constructor stub | |
25 | + } | |
26 | + | |
27 | + /** | |
28 | + * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) | |
29 | + */ | |
30 | + protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { | |
31 | + | |
32 | + response.getWriter().append("Served at: ").append(request.getContextPath()); | |
33 | + String id = request.getParameter("userId"); | |
34 | + String pw = request.getParameter("userPw"); | |
35 | + | |
36 | + PrintWriter out = response.getWriter(); | |
37 | + out.println("\n\n"+"Id : "+id); | |
38 | + out.println("\n"+"P/W : "+pw); | |
39 | + } | |
40 | + | |
41 | + /** | |
42 | + * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response) | |
43 | + */ | |
44 | + protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { | |
45 | + // TODO Auto-generated method stub | |
46 | + doGet(request, response); | |
47 | + } | |
48 | + | |
49 | +} |
+++ 202108/src/main/java/study/DB.java
... | ... | @@ -0,0 +1,32 @@ |
1 | +package study; | |
2 | + | |
3 | +import java.sql.Connection; | |
4 | +import java.sql.DriverManager; | |
5 | +import java.sql.PreparedStatement; | |
6 | +import java.sql.ResultSet; | |
7 | + | |
8 | +public class DB { | |
9 | + | |
10 | + // 데이터베이스 연결 관련 변수 선언 | |
11 | + Connection conn = null; | |
12 | + PreparedStatement pstmt = null; | |
13 | + ResultSet ps = null; | |
14 | + | |
15 | + // 데이터베이스 연결관련 정보를 문자열로 선언 | |
16 | + String driver = "org.mariadb.jdbc.Driver"; | |
17 | + String url = "jdbc:mariadb://localhost:3306/mydb"; | |
18 | + | |
19 | + | |
20 | + public void conn() { | |
21 | + try { | |
22 | + Class.forName(driver); | |
23 | + conn = DriverManager.getConnection(url, "root", "1234"); | |
24 | + System.out.println("Database Connect Success!!"); | |
25 | + } catch (Exception e) { | |
26 | + e.printStackTrace(); | |
27 | + System.out.println("Database Connects Fail!!"); | |
28 | + } | |
29 | + | |
30 | + } | |
31 | + | |
32 | +} |
+++ 202108/src/main/java/study/DBTest.java
... | ... | @@ -0,0 +1,43 @@ |
1 | +package study; | |
2 | + | |
3 | +import java.io.IOException; | |
4 | +import javax.servlet.ServletException; | |
5 | +import javax.servlet.annotation.WebServlet; | |
6 | +import javax.servlet.http.HttpServlet; | |
7 | +import javax.servlet.http.HttpServletRequest; | |
8 | +import javax.servlet.http.HttpServletResponse; | |
9 | + | |
10 | +/** | |
11 | + * Servlet implementation class DBTest | |
12 | + */ | |
13 | +@WebServlet("/DBTest") | |
14 | +public class DBTest extends HttpServlet { | |
15 | + private static final long serialVersionUID = 1L; | |
16 | + | |
17 | + /** | |
18 | + * @see HttpServlet#HttpServlet() | |
19 | + */ | |
20 | + public DBTest() { | |
21 | + super(); | |
22 | + // TODO Auto-generated constructor stub | |
23 | + } | |
24 | + | |
25 | + /** | |
26 | + * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) | |
27 | + */ | |
28 | + protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { | |
29 | + | |
30 | + DB db = new DB(); | |
31 | + db.conn(); | |
32 | + | |
33 | + } | |
34 | + | |
35 | + /** | |
36 | + * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response) | |
37 | + */ | |
38 | + protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { | |
39 | + // TODO Auto-generated method stub | |
40 | + doGet(request, response); | |
41 | + } | |
42 | + | |
43 | +} |
+++ 202108/src/main/webapp/0813/form.html
... | ... | @@ -0,0 +1,15 @@ |
1 | +<!DOCTYPE html> | |
2 | +<html> | |
3 | +<head> | |
4 | +<meta charset="UTF-8"> | |
5 | +<title>Insert title here</title> | |
6 | +</head> | |
7 | +<body> | |
8 | + <form action="/202108/Action"> | |
9 | + Id : <input type="text" name="userId"><br /> | |
10 | + P/W : <input type="password" name="userPw"><br /> | |
11 | + <input type="submit" value="Send"> | |
12 | + <input type="reset" value="Reset"> | |
13 | + </form> | |
14 | +</body> | |
15 | +</html>(No newline at end of file) |
+++ 202108/src/main/webapp/0817/forward_action.jsp
... | ... | @@ -0,0 +1,6 @@ |
1 | +<%@ page language="java" contentType="text/html; charset=UTF-8" | |
2 | + pageEncoding="UTF-8"%> | |
3 | +<% request.setCharacterEncoding("UTF-8"); %> | |
4 | +<jsp:forward page="response_result.jsp"> | |
5 | + <jsp:param value="010-1111-2222" name="tel"/> | |
6 | +</jsp:forward>(No newline at end of file) |
+++ 202108/src/main/webapp/0817/redirect_action.jsp
... | ... | @@ -0,0 +1,1 @@ |
1 | +<%response.sendRedirect("response_result.jsp"); %>(No newline at end of file) |
+++ 202108/src/main/webapp/0817/request.html
... | ... | @@ -0,0 +1,25 @@ |
1 | +<!DOCTYPE html> | |
2 | +<html lang="ko"> | |
3 | +<head> | |
4 | +<meta charset="UTF-8"> | |
5 | +<title>Insert title here</title> | |
6 | +</head> | |
7 | +<body> | |
8 | + <h2>Request Test Form</h2> | |
9 | + <form action="request.jsp" method="post"> | |
10 | + 이름 : <input type="text" name="username"><br /> | |
11 | + 직업 : <select name="job"> | |
12 | + <option value="무직">무직</option> | |
13 | + <option value="회사원">회사원</option> | |
14 | + <option value="전문직">전문직</option> | |
15 | + <option value="학생">학생</option> | |
16 | + </select><br /> | |
17 | + 관심분야 : | |
18 | + <label><input type="checkbox" name="favorite" value="정치"> 정치</label> | |
19 | + <label><input type="checkbox" name="favorite" value="사회"> 사회</label> | |
20 | + <label><input type="checkbox" name="favorite" value="정보통신"> 정보통신</label><br /><br /> | |
21 | + <input type="submit"value="확인"> | |
22 | + <input type="submit"value="취소"> | |
23 | + </form> | |
24 | +</body> | |
25 | +</html>(No newline at end of file) |
+++ 202108/src/main/webapp/0817/request.jsp
... | ... | @@ -0,0 +1,36 @@ |
1 | +<%@ page language="java" contentType="text/html; charset=UTF-8" | |
2 | + pageEncoding="UTF-8"%> | |
3 | +<% | |
4 | + request.setCharacterEncoding("utf-8"); | |
5 | + String name = request.getParameter("username"); | |
6 | + String job = request.getParameter("job"); | |
7 | + /* 다중 checkBox로 인한 변수 배열화 */ | |
8 | + String[] favorite = request.getParameterValues("favorite"); | |
9 | +%> | |
10 | +<!DOCTYPE html> | |
11 | +<html> | |
12 | +<head> | |
13 | +<meta charset="UTF-8"> | |
14 | +<title>Insert title here</title> | |
15 | +</head> | |
16 | +<body> | |
17 | + <h2>Request Test Form <em>client</em></h2> | |
18 | + <hr /> | |
19 | + <% | |
20 | + out.println("이름 : " + name + "<br>"); | |
21 | + out.println("직업 : " + job + "<br>"); | |
22 | + out.println("관심분야 :"); | |
23 | + for(int i = 0; i < favorite.length; i++){ | |
24 | + out.println(favorite[i] + ","); | |
25 | + } | |
26 | + %> | |
27 | + <br /><br /><br /> | |
28 | + Client IP Address : <%=request.getRemoteAddr() %> <br /> | |
29 | + Client IP Port : <%=request.getRemotePort() %> <br /> | |
30 | + Request Method : <%=request.getMethod() %> <br /> | |
31 | + Request Protocol : <%=request.getProtocol() %> <br /> | |
32 | + | |
33 | + | |
34 | + | |
35 | +</body> | |
36 | +</html>(No newline at end of file) |
+++ 202108/src/main/webapp/0817/response.jsp
... | ... | @@ -0,0 +1,20 @@ |
1 | +<%@ page language="java" contentType="text/html; charset=UTF-8" | |
2 | + pageEncoding="UTF-8"%> | |
3 | +<!DOCTYPE html> | |
4 | +<html> | |
5 | +<head> | |
6 | +<meta charset="UTF-8"> | |
7 | +<title>Response 내장 객체 Test</title> | |
8 | +</head> | |
9 | +<body> | |
10 | + <h2>Forward, sendRediect Test</h2> | |
11 | + <form action="forward_action.jsp" method="post"> | |
12 | + forward Action : <input type="text" name="username"> | |
13 | + <input type="submit" value="Send"> | |
14 | + </form> | |
15 | + <form action="redirect_action.jsp" method="post"> | |
16 | + sendRediect Action : <input type="text" name="username"> | |
17 | + <input type="submit" value="send"> | |
18 | + </form> | |
19 | +</body> | |
20 | +</html>(No newline at end of file) |
+++ 202108/src/main/webapp/0817/response_result.jsp
... | ... | @@ -0,0 +1,15 @@ |
1 | +<%@ page language="java" contentType="text/html; charset=UTF-8" | |
2 | + pageEncoding="UTF-8"%> | |
3 | +<!DOCTYPE html> | |
4 | +<html lang="ko"> | |
5 | +<head> | |
6 | +<meta charset="UTF-8"> | |
7 | +<title>Response Result print Page</title> | |
8 | +</head> | |
9 | +<body> | |
10 | + 지금 보이는 화면은 response_result.jsp에서 출력한 결과입니다. | |
11 | + <hr /> | |
12 | + 이름 : <%=request.getParameter("username") %> <br /> | |
13 | + 전화번호 : <%=request.getParameter("tel") %> | |
14 | +</body> | |
15 | +</html>(No newline at end of file) |
+++ 202108/src/main/webapp/0817/session.jsp
... | ... | @@ -0,0 +1,22 @@ |
1 | +<%@ page language="java" contentType="text/html; charset=UTF-8" | |
2 | + pageEncoding="UTF-8"%> | |
3 | +<!DOCTYPE html> | |
4 | +<html> | |
5 | +<head> | |
6 | +<meta charset="UTF-8"> | |
7 | +<title>Session 내장 객체 Test</title> | |
8 | +</head> | |
9 | +<body> | |
10 | + <h2>session 내장 객체</h2> | |
11 | + <hr /> | |
12 | + <% | |
13 | + if(session.isNew()){ | |
14 | + out.println("<script>alert('세션이 해제되어 다시 설정합니다.')</script>"); | |
15 | + session.setAttribute("login", "risker"); | |
16 | + } | |
17 | + %> | |
18 | + <%=session.getAttribute("login") %>님 환영합니다.!! <br /> | |
19 | + 세션 아이디 : <%=session.getId() %> <br /> | |
20 | + 세션 유지기간 : <%=session.getMaxInactiveInterval() %> | |
21 | +</body> | |
22 | +</html>(No newline at end of file) |
+++ 202108/src/main/webapp/WEB-INF/lib/mariadb-java-client-2.7.3.jar
Binary file is not shown |
+++ 202108/src/main/webapp/shopping/add.jsp
... | ... | @@ -0,0 +1,33 @@ |
1 | +<%@page import="java.util.ArrayList"%> | |
2 | +<%@ page language="java" contentType="text/html; charset=UTF-8" | |
3 | + pageEncoding="UTF-8"%> | |
4 | +<% | |
5 | + request.setCharacterEncoding("UTF-8"); | |
6 | + String product = request.getParameter("product"); | |
7 | + | |
8 | + /*세션에 저장되 있는 list가져오기*/ | |
9 | + ArrayList list = (ArrayList)session.getAttribute("productlist"); | |
10 | + /*Casting*/ | |
11 | + | |
12 | + /*만약 세션에 저장되 있는 list가 없다면 새로운 list를 세션에 저장*/ | |
13 | + if(list == null){ | |
14 | + list = new ArrayList(); /*새로운 list 저장*/ | |
15 | + session.setAttribute("productlist", list); //세션에 list 저장 | |
16 | + } | |
17 | + list.add(product); //list에 장바구니 물품 추가 | |
18 | +%> | |
19 | +<!DOCTYPE html> | |
20 | +<html> | |
21 | +<head> | |
22 | +<meta charset="UTF-8"> | |
23 | +<title>add Cart</title> | |
24 | +</head> | |
25 | +<body> | |
26 | + <script> | |
27 | + alert("<%=product %> was added!"); | |
28 | + | |
29 | + /*뒤로가기*/ | |
30 | + history.go(-1); | |
31 | + </script> | |
32 | +</body> | |
33 | +</html>(No newline at end of file) |
+++ 202108/src/main/webapp/shopping/check.jsp
... | ... | @@ -0,0 +1,33 @@ |
1 | +<%@page import="java.util.ArrayList"%> | |
2 | +<%@ page language="java" contentType="text/html; charset=UTF-8" | |
3 | + pageEncoding="UTF-8"%> | |
4 | +<% | |
5 | + | |
6 | +%> | |
7 | +<!DOCTYPE html> | |
8 | +<html> | |
9 | +<head> | |
10 | +<meta charset="UTF-8"> | |
11 | +<title>Cart List</title> | |
12 | +</head> | |
13 | +<body> | |
14 | + <h2>Calculating</h2> | |
15 | + selected Product List | |
16 | + <hr /> | |
17 | + <% | |
18 | + /* 세션에 저장되어 있는 productlist를 arraylist에 저장 */ | |
19 | + ArrayList list = (ArrayList)session.getAttribute("productlist"); | |
20 | + | |
21 | + /* 세션에 productlist가 없으며 */ | |
22 | + if(list == null){ | |
23 | + out.println("None selected product!!!"); | |
24 | + }else{ | |
25 | + | |
26 | + /* productname이라는 이름으로 list에서 값을 하나씩 꺼냄 */ | |
27 | + for(Object productname:list){ | |
28 | + out.println(productname+"<br>"); | |
29 | + } | |
30 | + } | |
31 | + %> | |
32 | +</body> | |
33 | +</html>(No newline at end of file) |
+++ 202108/src/main/webapp/shopping/login.jsp
... | ... | @@ -0,0 +1,16 @@ |
1 | +<%@ page language="java" contentType="text/html; charset=UTF-8" | |
2 | + pageEncoding="UTF-8"%> | |
3 | +<!DOCTYPE html> | |
4 | +<html> | |
5 | +<head> | |
6 | +<meta charset="UTF-8"> | |
7 | +<title>Login</title> | |
8 | +</head> | |
9 | +<body> | |
10 | + <h2>Login</h2> | |
11 | + <form action="selProduct.jsp" method="post"> | |
12 | + <input type="text" name="username"> | |
13 | + <input type="submit" value="Login"> | |
14 | + </form> | |
15 | +</body> | |
16 | +</html>(No newline at end of file) |
+++ 202108/src/main/webapp/shopping/selProduct.jsp
... | ... | @@ -0,0 +1,36 @@ |
1 | +<%@ page language="java" contentType="text/html; charset=UTF-8" | |
2 | + pageEncoding="UTF-8"%> | |
3 | +<% | |
4 | + request.setCharacterEncoding("UTF-8"); | |
5 | + session.setAttribute("username", request.getParameter("username")); | |
6 | +%> | |
7 | +<!DOCTYPE html> | |
8 | +<html> | |
9 | +<head> | |
10 | +<meta charset="UTF-8"> | |
11 | +<title>Product List</title> | |
12 | +<style> | |
13 | + #page {width: 720px; margin: 0 auto;} | |
14 | +</style> | |
15 | +</head> | |
16 | +<body> | |
17 | + <div id="page"> | |
18 | + <h2>Select Product</h2> | |
19 | + <hr /> | |
20 | + | |
21 | + <%=session.getAttribute("username") %> was login | |
22 | + <hr /> | |
23 | + <form action="add.jsp" method="post"> | |
24 | + <select name="product"> | |
25 | + <option value="hood">Hood</option> | |
26 | + <option value="jean">Jean</option> | |
27 | + <option value="coat">Coat</option> | |
28 | + <option value="padding">Padding</option> | |
29 | + <option value="short">Short</option> | |
30 | + </select> | |
31 | + <input type="submit" value="add Cart"> | |
32 | + </form> | |
33 | + <a href="check.jsp">Calculating</a> | |
34 | + </div> | |
35 | +</body> | |
36 | +</html>(No newline at end of file) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?