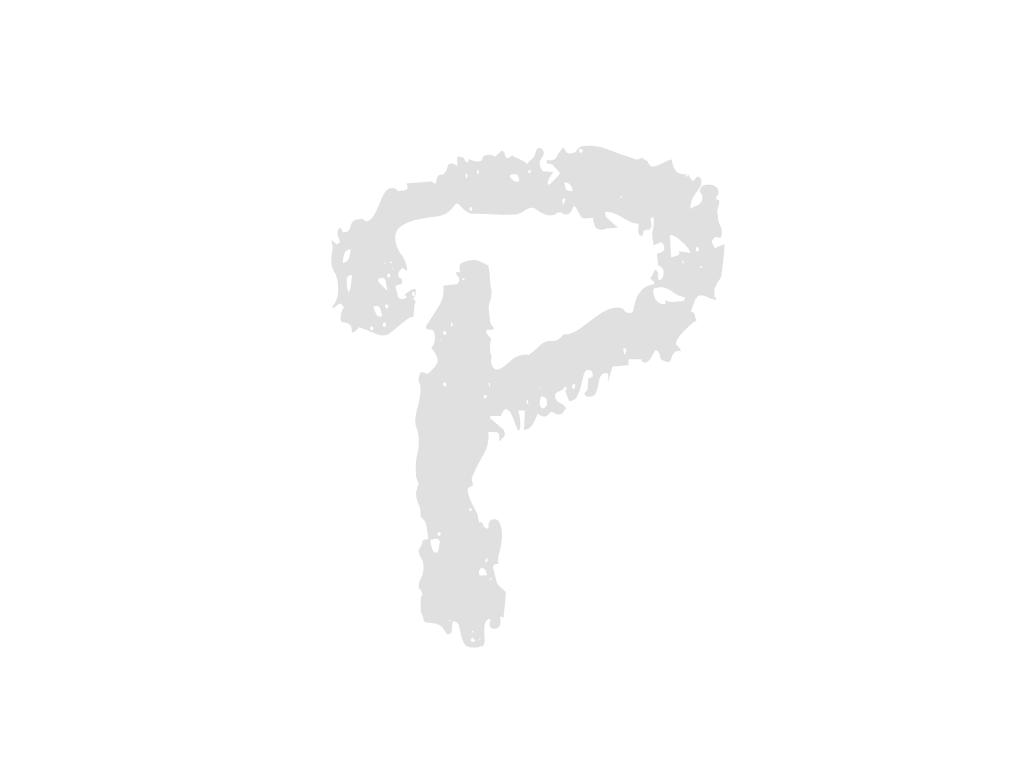
--- 202108/.settings/org.eclipse.wst.common.component
+++ 202108/.settings/org.eclipse.wst.common.component
... | ... | @@ -1,8 +1,15 @@ |
1 | 1 |
<?xml version="1.0" encoding="UTF-8"?><project-modules id="moduleCoreId" project-version="1.5.0"> |
2 |
+ |
|
2 | 3 |
<wb-module deploy-name="202108"> |
4 |
+ |
|
3 | 5 |
<wb-resource deploy-path="/" source-path="/src/main/webapp" tag="defaultRootSource"/> |
6 |
+ |
|
4 | 7 |
<wb-resource deploy-path="/WEB-INF/classes" source-path="/src/main/java"/> |
8 |
+ |
|
5 | 9 |
<property name="context-root" value="202108"/> |
10 |
+ |
|
6 | 11 |
<property name="java-output-path" value="/202108/build/classes"/> |
12 |
+ |
|
7 | 13 |
</wb-module> |
14 |
+ |
|
8 | 15 |
</project-modules> |
+++ 202108/src/main/java/cart/AddServer.java
... | ... | @@ -0,0 +1,64 @@ |
1 | +package cart; | |
2 | + | |
3 | +import java.io.IOException; | |
4 | +import java.io.PrintWriter; | |
5 | + | |
6 | +import javax.servlet.ServletException; | |
7 | +import javax.servlet.annotation.WebServlet; | |
8 | +import javax.servlet.http.HttpServlet; | |
9 | +import javax.servlet.http.HttpServletRequest; | |
10 | +import javax.servlet.http.HttpServletResponse; | |
11 | + | |
12 | +/** | |
13 | + * Servlet implementation class AddServer | |
14 | + */ | |
15 | +@WebServlet("/AddServer") | |
16 | +public class AddServer extends HttpServlet { | |
17 | + private static final long serialVersionUID = 1L; | |
18 | + | |
19 | + /** | |
20 | + * @see HttpServlet#HttpServlet() | |
21 | + */ | |
22 | + public AddServer() { | |
23 | + super(); | |
24 | + // TODO Auto-generated constructor stub | |
25 | + } | |
26 | + | |
27 | + /** | |
28 | + * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) | |
29 | + */ | |
30 | + protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { | |
31 | + | |
32 | + doPost(request, response); | |
33 | + | |
34 | + } | |
35 | + | |
36 | + /** | |
37 | + * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response) | |
38 | + */ | |
39 | + protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { | |
40 | + | |
41 | + request.setCharacterEncoding("UTF-8"); | |
42 | + response.setContentType("text/html; charset=utf-8"); | |
43 | + | |
44 | + String item = request.getParameter("product"); | |
45 | + int no = Integer.parseInt(request.getParameter("no")); | |
46 | + | |
47 | + CartDAO dao = new CartDAO(); | |
48 | + dao.setCart(no, item); | |
49 | + PrintWriter out = response.getWriter(); | |
50 | + out.println("<script>alert('The item was added!')</script>"); | |
51 | + out.println("<script>history.go(-1)</script>"); | |
52 | + | |
53 | + | |
54 | + } | |
55 | + | |
56 | +} | |
57 | + | |
58 | + | |
59 | + | |
60 | + | |
61 | + | |
62 | + | |
63 | + | |
64 | + |
+++ 202108/src/main/java/cart/CartDAO.java
... | ... | @@ -0,0 +1,67 @@ |
1 | +package cart; | |
2 | + | |
3 | +import java.sql.Connection; | |
4 | +import java.sql.DriverManager; | |
5 | +import java.sql.PreparedStatement; | |
6 | +import java.sql.ResultSet; | |
7 | + | |
8 | +public class CartDAO { | |
9 | + | |
10 | + // 데이터베이스 연결 관련 변수 선언 | |
11 | + Connection conn = null; //프로그램과 데이터베이스를 연결 | |
12 | + PreparedStatement pstmt = null; // //쿼리를 실행 | |
13 | + ResultSet rs = null; // 쿼리 실행결과를 담음 | |
14 | + | |
15 | + // 데이터베이스 연결관련 정보를 문자열로 선언 | |
16 | + String driver = "com.mysql.jdbc.Driver"; | |
17 | + String url = "jdbc:mysql://db.asin21.com:3306/db_demo07"; | |
18 | + | |
19 | + | |
20 | + public void conn() { | |
21 | + try { | |
22 | + Class.forName(driver); | |
23 | + conn = DriverManager.getConnection(url, "demo07", "demo07!"); | |
24 | + System.out.println("Database Connect Success!!"); | |
25 | + } catch (Exception e) { | |
26 | + e.printStackTrace(); | |
27 | + System.out.println("Database Connect Fail!!"); | |
28 | + } | |
29 | + | |
30 | + } | |
31 | + public void disconn() { | |
32 | + try { | |
33 | + if(rs != null) { | |
34 | + rs.close(); | |
35 | + } | |
36 | + if(pstmt != null) { | |
37 | + pstmt.close(); | |
38 | + } | |
39 | + if(conn != null) { | |
40 | + conn.close(); | |
41 | + } | |
42 | + } catch (Exception e) { | |
43 | + e.printStackTrace(); | |
44 | + } | |
45 | + } | |
46 | + | |
47 | + public void setCart(int member_no, String product_name) { | |
48 | + conn(); | |
49 | + | |
50 | + try { | |
51 | + pstmt = conn.prepareStatement("insert into cart values(null, ?, ?)"); | |
52 | + pstmt.setInt(1, member_no); | |
53 | + pstmt.setString(2, product_name); | |
54 | + pstmt.executeUpdate(); | |
55 | + | |
56 | + | |
57 | + } catch (Exception e) { | |
58 | + e.printStackTrace(); | |
59 | + } | |
60 | + disconn(); | |
61 | + } | |
62 | + | |
63 | +} | |
64 | + | |
65 | + | |
66 | + | |
67 | + |
+++ 202108/src/main/java/cart/CartDTO.java
... | ... | @@ -0,0 +1,29 @@ |
1 | +package cart; | |
2 | + | |
3 | +public class CartDTO { | |
4 | + | |
5 | + int no; | |
6 | + int member_no; | |
7 | + String product_name; | |
8 | + public int getNo() { | |
9 | + return no; | |
10 | + } | |
11 | + public void setNo(int no) { | |
12 | + this.no = no; | |
13 | + } | |
14 | + | |
15 | + public int getMember_no() { | |
16 | + return member_no; | |
17 | + } | |
18 | + public void setMember_no(int member_no) { | |
19 | + this.member_no = member_no; | |
20 | + } | |
21 | + | |
22 | + public String getProduct_name() { | |
23 | + return product_name; | |
24 | + } | |
25 | + public void setProduct_name(String product_name) { | |
26 | + this.product_name = product_name; | |
27 | + } | |
28 | + | |
29 | +} |
+++ 202108/src/main/java/cart/LoginDAO.java
... | ... | @@ -0,0 +1,101 @@ |
1 | +package cart; | |
2 | + | |
3 | +import java.sql.Connection; | |
4 | +import java.sql.DriverManager; | |
5 | +import java.sql.PreparedStatement; | |
6 | +import java.sql.ResultSet; | |
7 | + | |
8 | +public class LoginDAO { | |
9 | + | |
10 | + // 데이터베이스 연결 관련 변수 선언 | |
11 | + Connection conn = null; //프로그램과 데이터베이스를 연결 | |
12 | + PreparedStatement pstmt = null; // //쿼리를 실행 | |
13 | + ResultSet rs = null; // 쿼리 실행결과를 담음 | |
14 | + | |
15 | + // 데이터베이스 연결관련 정보를 문자열로 선언 | |
16 | + String driver = "com.mysql.jdbc.Driver"; | |
17 | + String url = "jdbc:mysql://db.asin21.com:3306/db_demo07"; | |
18 | + | |
19 | + | |
20 | + public void conn() { | |
21 | + try { | |
22 | + Class.forName(driver); | |
23 | + conn = DriverManager.getConnection(url, "demo07", "demo07!"); | |
24 | + System.out.println("Database Connect Success!!"); | |
25 | + } catch (Exception e) { | |
26 | + e.printStackTrace(); | |
27 | + System.out.println("Database Connect Fail!!"); | |
28 | + } | |
29 | + | |
30 | + } | |
31 | + public void disconn() { | |
32 | + try { | |
33 | + if(rs != null) { | |
34 | + rs.close(); | |
35 | + } | |
36 | + if(pstmt != null) { | |
37 | + pstmt.close(); | |
38 | + } | |
39 | + if(conn != null) { | |
40 | + conn.close(); | |
41 | + } | |
42 | + } catch (Exception e) { | |
43 | + e.printStackTrace(); | |
44 | + } | |
45 | + } | |
46 | + | |
47 | + //login function | |
48 | + public boolean getLogin(String userid, String userpw) { | |
49 | + LoginDTO login = new LoginDTO(); | |
50 | + conn(); | |
51 | + | |
52 | + try { | |
53 | + pstmt = conn.prepareStatement("select * from member where id=? and pw=?"); | |
54 | + pstmt.setString(1, userid); | |
55 | + pstmt.setString(2, userpw); | |
56 | + rs = pstmt.executeQuery(); | |
57 | + rs.next(); | |
58 | + | |
59 | + login.setNo(rs.getInt("no")); | |
60 | + login.setId(rs.getString("id")); | |
61 | + login.setPw(rs.getString("pw")); | |
62 | + return true; | |
63 | + | |
64 | + } catch (Exception e) { | |
65 | + System.out.println(e); | |
66 | + disconn(); | |
67 | + return false; | |
68 | + } | |
69 | + | |
70 | + } | |
71 | + | |
72 | + //getting no function | |
73 | + public int getMemberNo(String userid, String userpw) { | |
74 | + int result = 0; | |
75 | + conn(); | |
76 | + | |
77 | + try { | |
78 | + pstmt = conn.prepareStatement("select no from member where id=? and pw=?"); | |
79 | + pstmt.setString(1, userid); | |
80 | + pstmt.setString(2, userpw); | |
81 | + rs = pstmt.executeQuery(); | |
82 | + rs.next(); | |
83 | + result = rs.getInt("no"); | |
84 | + } catch (Exception e) { | |
85 | + e.printStackTrace(); | |
86 | + } | |
87 | + disconn(); | |
88 | + return result; | |
89 | + } | |
90 | + | |
91 | +} | |
92 | + | |
93 | + | |
94 | + | |
95 | + | |
96 | + | |
97 | + | |
98 | + | |
99 | + | |
100 | + | |
101 | + |
+++ 202108/src/main/java/cart/LoginDTO.java
... | ... | @@ -0,0 +1,31 @@ |
1 | +package cart; | |
2 | + | |
3 | +public class LoginDTO { | |
4 | + | |
5 | + int no; | |
6 | + String id; | |
7 | + String pw; | |
8 | + public int getNo() { | |
9 | + return no; | |
10 | + } | |
11 | + public void setNo(int no) { | |
12 | + this.no = no; | |
13 | + } | |
14 | + | |
15 | + public String getId() { | |
16 | + return id; | |
17 | + } | |
18 | + public void setId(String id) { | |
19 | + this.id = id; | |
20 | + } | |
21 | + | |
22 | + public String getPw() { | |
23 | + return pw; | |
24 | + } | |
25 | + public void setPw(String pw) { | |
26 | + this.pw = pw; | |
27 | + } | |
28 | + | |
29 | + | |
30 | + | |
31 | +} |
+++ 202108/src/main/java/cart/LoginServer.java
... | ... | @@ -0,0 +1,73 @@ |
1 | +package cart; | |
2 | + | |
3 | +import java.io.IOException; | |
4 | +import javax.servlet.ServletException; | |
5 | +import javax.servlet.annotation.WebServlet; | |
6 | +import javax.servlet.http.HttpServlet; | |
7 | +import javax.servlet.http.HttpServletRequest; | |
8 | +import javax.servlet.http.HttpServletResponse; | |
9 | +import javax.servlet.http.HttpSession; | |
10 | + | |
11 | +/** | |
12 | + * Servlet implementation class LoginServer | |
13 | + */ | |
14 | +@WebServlet("/LoginServer") | |
15 | +public class LoginServer extends HttpServlet { | |
16 | + private static final long serialVersionUID = 1L; | |
17 | + | |
18 | + /** | |
19 | + * @see HttpServlet#HttpServlet() | |
20 | + */ | |
21 | + public LoginServer() { | |
22 | + super(); | |
23 | + // TODO Auto-generated constructor stub | |
24 | + } | |
25 | + | |
26 | + /** | |
27 | + * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) | |
28 | + */ | |
29 | + protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { | |
30 | + | |
31 | + doPost(request, response); | |
32 | + | |
33 | + } | |
34 | + | |
35 | + /** | |
36 | + * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response) | |
37 | + */ | |
38 | + protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { | |
39 | + | |
40 | + request.setCharacterEncoding("UTF-8"); | |
41 | + LoginDTO login = new LoginDTO(); | |
42 | + boolean result; | |
43 | + | |
44 | + String userid = request.getParameter("userid"); | |
45 | + String userpw = request.getParameter("userpw"); | |
46 | + | |
47 | + LoginDAO dao = new LoginDAO(); | |
48 | + result= dao.getLogin(userid, userpw); | |
49 | + | |
50 | + int userNo = dao.getMemberNo(userid, userpw); | |
51 | + | |
52 | + if(result) {//login success | |
53 | + response.sendRedirect("cart/selProduct.jsp"); | |
54 | + HttpSession session = request.getSession(); | |
55 | + session.setAttribute("no", userNo); | |
56 | + }else { | |
57 | + response.sendRedirect("cart/login.jsp"); | |
58 | + } | |
59 | + } | |
60 | + | |
61 | +} | |
62 | + | |
63 | + | |
64 | + | |
65 | + | |
66 | + | |
67 | + | |
68 | + | |
69 | + | |
70 | + | |
71 | + | |
72 | + | |
73 | + |
+++ 202108/src/main/java/cart/ProductDAO.java
... | ... | @@ -0,0 +1,93 @@ |
1 | +package cart; | |
2 | + | |
3 | +import java.sql.Connection; | |
4 | +import java.sql.DriverManager; | |
5 | +import java.sql.PreparedStatement; | |
6 | +import java.sql.ResultSet; | |
7 | +import java.util.ArrayList; | |
8 | + | |
9 | +import javax.print.attribute.standard.PrinterState; | |
10 | + | |
11 | +public class ProductDAO { | |
12 | + | |
13 | + // 데이터베이스 연결 관련 변수 선언 | |
14 | + Connection conn = null; //프로그램과 데이터베이스를 연결 | |
15 | + PreparedStatement pstmt = null; // //쿼리를 실행 | |
16 | + ResultSet rs = null; // 쿼리 실행결과를 담음 | |
17 | + | |
18 | + // 데이터베이스 연결관련 정보를 문자열로 선언 | |
19 | + String driver = "com.mysql.jdbc.Driver"; | |
20 | + String url = "jdbc:mysql://db.asin21.com:3306/db_demo07"; | |
21 | + | |
22 | + | |
23 | + public void conn() { | |
24 | + try { | |
25 | + Class.forName(driver); | |
26 | + conn = DriverManager.getConnection(url, "demo07", "demo07!"); | |
27 | + System.out.println("Database Connect Success!!"); | |
28 | + } catch (Exception e) { | |
29 | + e.printStackTrace(); | |
30 | + System.out.println("Database Connect Fail!!"); | |
31 | + } | |
32 | + | |
33 | + } | |
34 | + public void disconn() { | |
35 | + try { | |
36 | + if(rs != null) { | |
37 | + rs.close(); | |
38 | + } | |
39 | + if(pstmt != null) { | |
40 | + pstmt.close(); | |
41 | + } | |
42 | + if(conn != null) { | |
43 | + conn.close(); | |
44 | + } | |
45 | + } catch (Exception e) { | |
46 | + e.printStackTrace(); | |
47 | + } | |
48 | + } | |
49 | + | |
50 | + public ArrayList<ProductDTO> getProductList(){ | |
51 | + ArrayList<ProductDTO> list = new ArrayList<ProductDTO>(); | |
52 | + conn(); | |
53 | + | |
54 | + try { | |
55 | + pstmt = conn.prepareStatement("select * from product"); | |
56 | + rs = pstmt.executeQuery(); | |
57 | + | |
58 | + while(rs.next()) { | |
59 | + ProductDTO product = new ProductDTO(); | |
60 | + product.setNo(rs.getInt("no")); | |
61 | + product.setName(rs.getString("name")); | |
62 | + product.setPrice(rs.getString("price")); | |
63 | + | |
64 | + list.add(product); | |
65 | + } | |
66 | + | |
67 | + } catch (Exception e) { | |
68 | + e.printStackTrace(); | |
69 | + } | |
70 | + disconn(); | |
71 | + return list; | |
72 | + } | |
73 | + | |
74 | + | |
75 | + | |
76 | +} | |
77 | + | |
78 | + | |
79 | + | |
80 | + | |
81 | + | |
82 | + | |
83 | + | |
84 | + | |
85 | + | |
86 | + | |
87 | + | |
88 | + | |
89 | + | |
90 | + | |
91 | + | |
92 | + | |
93 | + |
+++ 202108/src/main/java/cart/ProductDTO.java
... | ... | @@ -0,0 +1,31 @@ |
1 | +package cart; | |
2 | + | |
3 | +public class ProductDTO { | |
4 | + | |
5 | + int no; | |
6 | + String name; | |
7 | + String price; | |
8 | + | |
9 | + public int getNo() { | |
10 | + return no; | |
11 | + } | |
12 | + public void setNo(int no) { | |
13 | + this.no = no; | |
14 | + } | |
15 | + | |
16 | + public String getName() { | |
17 | + return name; | |
18 | + } | |
19 | + public void setName(String name) { | |
20 | + this.name = name; | |
21 | + } | |
22 | + | |
23 | + public String getPrice() { | |
24 | + return price; | |
25 | + } | |
26 | + public void setPrice(String price) { | |
27 | + this.price = price; | |
28 | + } | |
29 | + | |
30 | + | |
31 | +} |
--- 202108/src/main/java/study/DB.java
+++ 202108/src/main/java/study/DB.java
... | ... | @@ -8,9 +8,9 @@ |
8 | 8 |
public class DB { |
9 | 9 |
|
10 | 10 |
// 데이터베이스 연결 관련 변수 선언 |
11 |
- Connection conn = null; |
|
12 |
- PreparedStatement pstmt = null; |
|
13 |
- ResultSet ps = null; |
|
11 |
+ Connection conn = null; //프로그램과 데이터베이스를 연결 |
|
12 |
+ PreparedStatement pstmt = null; // //쿼리를 실행 |
|
13 |
+ ResultSet rs = null; // 쿼리 실행결과를 담음 |
|
14 | 14 |
|
15 | 15 |
// 데이터베이스 연결관련 정보를 문자열로 선언 |
16 | 16 |
String driver = "com.mysql.jdbc.Driver"; |
... | ... | @@ -28,5 +28,42 @@ |
28 | 28 |
} |
29 | 29 |
|
30 | 30 |
} |
31 |
+ public void disconn() { |
|
32 |
+ try { |
|
33 |
+ if(rs != null) { |
|
34 |
+ rs.close(); |
|
35 |
+ } |
|
36 |
+ if(pstmt != null) { |
|
37 |
+ pstmt.close(); |
|
38 |
+ } |
|
39 |
+ if(conn != null) { |
|
40 |
+ conn.close(); |
|
41 |
+ } |
|
42 |
+ } catch (Exception e) { |
|
43 |
+ e.printStackTrace(); |
|
44 |
+ } |
|
45 |
+ } |
|
46 |
+ |
|
47 |
+ //select Test |
|
48 |
+ public String getLogin() { |
|
49 |
+ conn(); |
|
50 |
+ String id = ""; |
|
51 |
+ try { |
|
52 |
+ pstmt = conn.prepareStatement("select id from login"); |
|
53 |
+ rs = pstmt.executeQuery(); |
|
54 |
+ rs.next(); |
|
55 |
+ id = rs.getString("id"); |
|
56 |
+ |
|
57 |
+ } catch (Exception e) { |
|
58 |
+ e.printStackTrace(); |
|
59 |
+ } |
|
60 |
+ disconn(); |
|
61 |
+ return id; |
|
62 |
+ } |
|
31 | 63 |
|
32 | 64 |
} |
65 |
+ |
|
66 |
+ |
|
67 |
+ |
|
68 |
+ |
|
69 |
+ |
--- 202108/src/main/java/study/DBTest.java
+++ 202108/src/main/java/study/DBTest.java
... | ... | @@ -28,7 +28,7 @@ |
28 | 28 |
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { |
29 | 29 |
|
30 | 30 |
DB db = new DB(); |
31 |
- db.conn(); |
|
31 |
+ System.out.println(db.getLogin()); |
|
32 | 32 |
|
33 | 33 |
} |
34 | 34 |
|
+++ 202108/src/main/webapp/cart/login.jsp
... | ... | @@ -0,0 +1,17 @@ |
1 | +<%@ page language="java" contentType="text/html; charset=UTF-8" | |
2 | + pageEncoding="UTF-8"%> | |
3 | +<!DOCTYPE html> | |
4 | +<html> | |
5 | +<head> | |
6 | +<meta charset="UTF-8"> | |
7 | +<title>Login</title> | |
8 | +</head> | |
9 | +<body> | |
10 | + <h2><em style="color: red;">Login</em></h2> | |
11 | + <form action="/202108/LoginServer" method="post"> | |
12 | + ID : <input type="text" name="userid"> <br /> | |
13 | + P/W : <input type="password" name="userpw"> <br /> | |
14 | + <input type="submit" value="Login"> | |
15 | + </form> | |
16 | +</body> | |
17 | +</html>(No newline at end of file) |
+++ 202108/src/main/webapp/cart/selProduct.jsp
... | ... | @@ -0,0 +1,39 @@ |
1 | +<%@page import="cart.ProductDTO"%> | |
2 | +<%@page import="java.util.ArrayList"%> | |
3 | +<%@page import="cart.ProductDAO"%> | |
4 | +<%@ page language="java" contentType="text/html; charset=UTF-8" | |
5 | + pageEncoding="UTF-8"%> | |
6 | +<% | |
7 | + request.setCharacterEncoding("UTF-8"); | |
8 | + ProductDAO dao = new ProductDAO(); | |
9 | + ArrayList<ProductDTO> list = new ArrayList<ProductDTO>(); | |
10 | + list = dao.getProductList(); | |
11 | +%> | |
12 | +<!DOCTYPE html> | |
13 | +<html> | |
14 | +<head> | |
15 | +<meta charset="UTF-8"> | |
16 | +<title>Product List</title> | |
17 | +<style> | |
18 | + #page {width: 720px; margin: 0 auto;} | |
19 | +</style> | |
20 | +</head> | |
21 | +<body> | |
22 | + <div id="page"> | |
23 | + <h2>Select Product</h2> | |
24 | + <hr /> | |
25 | + <form action="/202108/AddServer" method="post"> | |
26 | + <input type="hidden" value="<%=session.getAttribute("no") %>" name="no"> | |
27 | + <select name="product"> | |
28 | + <% | |
29 | + for(ProductDTO item : list){ | |
30 | + out.println("<option>" + item.getName() + "</option>"); | |
31 | + } | |
32 | + %> | |
33 | + </select> | |
34 | + <input type="submit" value="add Cart"> | |
35 | + </form> | |
36 | + <a href="check.jsp">Calculating</a> | |
37 | + </div> | |
38 | +</body> | |
39 | +</html>(No newline at end of file) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?