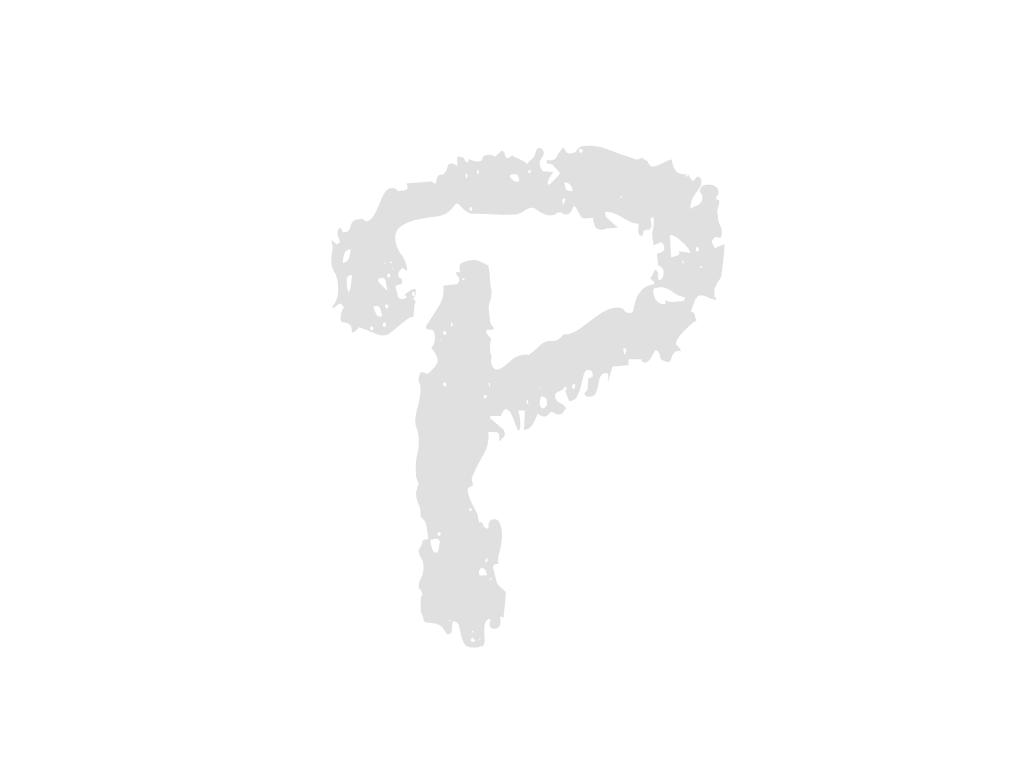
--- 20220126/DB.php
+++ 20220126/DB.php
... | ... | @@ -10,6 +10,7 @@ |
10 | 10 |
public function __construct(){ |
11 | 11 |
try { |
12 | 12 |
$this->db = new PDO("mysql:host=localhost;dbname=study", $this->user, $this->password, $this->option); |
13 |
+ $this->db->exec("set names UTF8"); |
|
13 | 14 |
}catch (PDOException $e){ |
14 | 15 |
echo "<h2>Database Connection FAIL!!</h2>"; |
15 | 16 |
echo "Because : " . $e . "<hr>"; |
... | ... | @@ -19,4 +20,53 @@ |
19 | 20 |
public function getDB() { |
20 | 21 |
return $this->db; |
21 | 22 |
} |
22 |
-}(No newline at end of file) |
|
23 |
+ |
|
24 |
+ public function selectTest() { |
|
25 |
+ try { |
|
26 |
+ $stmt = $this->db->prepare("select * from address where name = ? limit 1"); |
|
27 |
+ $stmt->execute(array("안효원")); |
|
28 |
+ $result = $stmt->fetchAll(); |
|
29 |
+ return $result; |
|
30 |
+ } catch (PDOException $e){ |
|
31 |
+ return $e; |
|
32 |
+ } |
|
33 |
+ } |
|
34 |
+ |
|
35 |
+ public function insertTest($name, $age, $tel, $area, $birth) { |
|
36 |
+ try { |
|
37 |
+ $stmt = $this->db->prepare("insert into address values(NULL, ?, ?, ?, ?, ?)"); |
|
38 |
+ $stmt->execute(array($name, $age, $tel, $area, $birth)); |
|
39 |
+ return true; |
|
40 |
+ }catch (PDOException $e) { |
|
41 |
+ echo $e; |
|
42 |
+ return false; |
|
43 |
+ } |
|
44 |
+ } |
|
45 |
+ |
|
46 |
+ public function updateTest($name) { |
|
47 |
+ try { |
|
48 |
+ $stmt = $this->db->prepare("update address set name=? where name='안효원'"); |
|
49 |
+ $stmt->execute(array($name)); |
|
50 |
+ return true; |
|
51 |
+ }catch (PDOException $e) { |
|
52 |
+ echo $e; |
|
53 |
+ return false; |
|
54 |
+ } |
|
55 |
+ } |
|
56 |
+ |
|
57 |
+ public function deleteTest($name) { |
|
58 |
+ try { |
|
59 |
+ $stmt = $this->db->prepare("delete from address where name=?"); |
|
60 |
+ $stmt->execute(array($name)); |
|
61 |
+ return true; |
|
62 |
+ }catch (PDOException $e){ |
|
63 |
+ echo $e; |
|
64 |
+ return false; |
|
65 |
+ } |
|
66 |
+ } |
|
67 |
+ |
|
68 |
+} |
|
69 |
+ |
|
70 |
+ |
|
71 |
+ |
|
72 |
+ |
--- 20220126/dbtest2.php
+++ 20220126/dbtest2.php
... | ... | @@ -2,4 +2,43 @@ |
2 | 2 |
require_once("DB.php"); |
3 | 3 |
|
4 | 4 |
$db = new DB(); |
5 |
- $db = $db->getDB();(No newline at end of file) |
|
5 |
+ |
|
6 |
+ echo "Select Test <br>"; |
|
7 |
+ $list = $db->selectTest(); |
|
8 |
+ |
|
9 |
+ print_r($list); |
|
10 |
+ |
|
11 |
+ |
|
12 |
+ echo "<br><hr>Insert Test<br>"; |
|
13 |
+ $result = $db->insertTest("안효원", 26, "010-5635-5918", "강원", "1998-08-07"); |
|
14 |
+ if($result) { |
|
15 |
+ echo "Insert Success!"; |
|
16 |
+ }else { |
|
17 |
+ echo "Insert Fail!"; |
|
18 |
+ } |
|
19 |
+ |
|
20 |
+ |
|
21 |
+ echo "<br><hr>Update Test<br>"; |
|
22 |
+ $result = $db->updateTest("김현식"); |
|
23 |
+ if($result) { |
|
24 |
+ echo "update Success!"; |
|
25 |
+ }else { |
|
26 |
+ echo "update Fail!"; |
|
27 |
+ } |
|
28 |
+ |
|
29 |
+ |
|
30 |
+ echo "<br><hr>Delete Test<br>"; |
|
31 |
+ $result = $db->deleteTest("안효원"); |
|
32 |
+ if($result) { |
|
33 |
+ echo "delete Success!"; |
|
34 |
+ }else { |
|
35 |
+ echo "delete Fail!"; |
|
36 |
+ } |
|
37 |
+ |
|
38 |
+ |
|
39 |
+ |
|
40 |
+ |
|
41 |
+ |
|
42 |
+ |
|
43 |
+ |
|
44 |
+ |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?