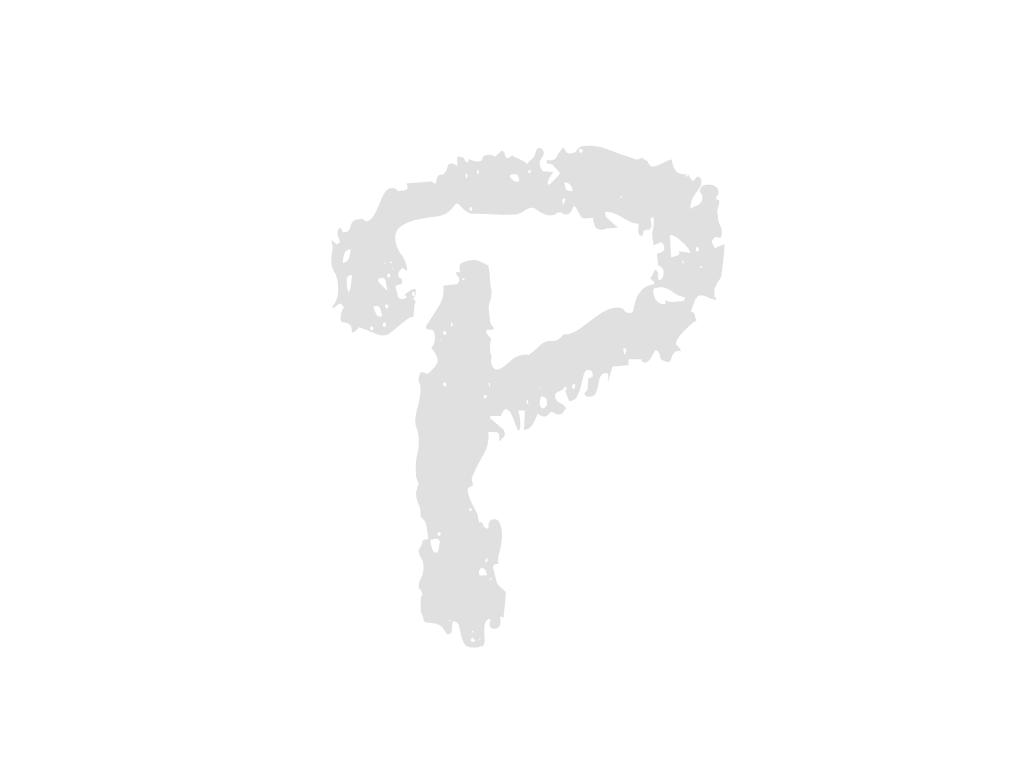
+++ 20220126/Address.php
... | ... | @@ -0,0 +1,60 @@ |
1 | +<?php | |
2 | + require_once("DB.php"); | |
3 | + | |
4 | + | |
5 | +class Address | |
6 | +{ | |
7 | + private $db; | |
8 | + | |
9 | + // address 인스턴스를 생성하면 자동으로 DB 연결 | |
10 | + public function __construct() { | |
11 | + $db = new DB(); | |
12 | + $this->db = $db->getDB(); | |
13 | + } | |
14 | + | |
15 | + public function getList() { | |
16 | + try { | |
17 | + $stmt = $this->db->prepare("select * from address"); | |
18 | + $stmt->execute(); | |
19 | + $result = $stmt->fetchAll(); | |
20 | + return $result; | |
21 | + }catch (PDOException $e) { | |
22 | + return $e; | |
23 | + } | |
24 | + } | |
25 | + | |
26 | + public function insertTest($name, $age, $tel, $area, $birth) { | |
27 | + try { | |
28 | + $stmt = $this->db->prepare("insert into address values(NULL, ?, ?, ?, ?, ?)"); | |
29 | + $stmt->execute(array($name, $age, $tel, $area, $birth)); | |
30 | + return true; | |
31 | + }catch (PDOException $e) { | |
32 | + echo $e; | |
33 | + return false; | |
34 | + } | |
35 | + } | |
36 | + | |
37 | + public function updateTest($no, $name, $age, $tel, $area, $birth) { | |
38 | + try { | |
39 | + $stmt = $this->db->prepare("update address set name=?, age=?, tel=?, area=?, birth=? where no=?"); | |
40 | + $stmt->execute(array($no)); | |
41 | + return true; | |
42 | + }catch (PDOException $e) { | |
43 | + echo $e; | |
44 | + return false; | |
45 | + } | |
46 | + } | |
47 | + | |
48 | + public function deleteTest($no) { | |
49 | + try { | |
50 | + $stmt = $this->db->prepare("delete from address where no=?"); | |
51 | + $stmt->execute(array($no)); | |
52 | + return true; | |
53 | + }catch (PDOException $e){ | |
54 | + echo $e; | |
55 | + return false; | |
56 | + } | |
57 | + } | |
58 | + | |
59 | + | |
60 | +}(No newline at end of file) |
--- 20220126/DB.php
+++ 20220126/DB.php
... | ... | @@ -43,10 +43,10 @@ |
43 | 43 |
} |
44 | 44 |
} |
45 | 45 |
|
46 |
- public function updateTest($name) { |
|
46 |
+ public function updateTest($name, $where) { |
|
47 | 47 |
try { |
48 |
- $stmt = $this->db->prepare("update address set name=? where name='안효원'"); |
|
49 |
- $stmt->execute(array($name)); |
|
48 |
+ $stmt = $this->db->prepare("update address set name=? where name=?"); |
|
49 |
+ $stmt->execute(array($name, $where)); |
|
50 | 50 |
return true; |
51 | 51 |
}catch (PDOException $e) { |
52 | 52 |
echo $e; |
+++ 20220126/address_delete.php
... | ... | @@ -0,0 +1,16 @@ |
1 | +<?php | |
2 | + require_once("Address.php"); | |
3 | + | |
4 | + $no = $_GET['no']; | |
5 | + | |
6 | + $address = new Address(); | |
7 | + | |
8 | + $result = $address->deleteTest($no); | |
9 | + if($result) { | |
10 | + echo "<script>alert('Delete Success!!!')</script>"; | |
11 | + echo "<script>document.location.href='address_view.php'</script>"; | |
12 | + }else { | |
13 | + echo "<script>alert('Delete Fail!!!')</script>"; | |
14 | + echo "<script>document.location.href='address_view.php'</script>"; | |
15 | + } | |
16 | + |
+++ 20220126/address_insert.php
... | ... | @@ -0,0 +1,23 @@ |
1 | +<?php | |
2 | + require_once("Address.php"); | |
3 | + | |
4 | + $name = $_POST['name']; | |
5 | + $age = $_POST['age']; | |
6 | + $tel = $_POST['tel']; | |
7 | + $area = $_POST['area']; | |
8 | + $birth = $_POST['birth']; | |
9 | + | |
10 | + $address = new Address(); | |
11 | + $result = $address->insertTest($name, $age, $tel, $area, $birth); | |
12 | + | |
13 | + if($result) { | |
14 | + echo "<script>alert('Insert Success!!')</script>"; | |
15 | + echo "<script>document.location.href='address_view.php'</script>"; | |
16 | + }else { | |
17 | + echo "<script>alert('Insert Fail!!')</script>"; | |
18 | + echo "<script>document.location.href='address_view.php'</script>"; | |
19 | + } | |
20 | + | |
21 | + | |
22 | + | |
23 | + |
+++ 20220126/address_update_back.php
... | ... | @@ -0,0 +1,19 @@ |
1 | +<?php | |
2 | + require_once("Address.php"); | |
3 | + $address = new Address(); | |
4 | + | |
5 | + $no = $_GET['no']; | |
6 | + $name = $_POST['name']; | |
7 | + $age = $_POST['age']; | |
8 | + $tel = $_POST['tel']; | |
9 | + $area = $_POST['area']; | |
10 | + $birth = $_POST['birth']; | |
11 | + | |
12 | + $result = $address->updateTest($no, $name, $age, $tel, $area, $birth); | |
13 | + if($result) { | |
14 | + echo "<script>alert('Update Success!!')</script>"; | |
15 | + echo "<script>document.location.href='address_view.php'</script>"; | |
16 | + }else { | |
17 | + echo "<script>alert('Update Fail!!')</script>"; | |
18 | + echo "<script>document.location.href='address_update_front.php?no=$no'</script>"; | |
19 | + } |
+++ 20220126/address_update_front.php
... | ... | @@ -0,0 +1,38 @@ |
1 | +<!doctype html> | |
2 | +<html lang="ko"> | |
3 | +<head> | |
4 | + <meta charset="UTF-8"> | |
5 | + <meta name="viewport" | |
6 | + content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> | |
7 | + <meta http-equiv="X-UA-Compatible" content="ie=edge"> | |
8 | + <title>Document</title> | |
9 | +</head> | |
10 | +<body> | |
11 | + <form action="address_update_back.php?no=<?php echo $_GET['no'] ?>" method="post"> | |
12 | + <label for="name"> | |
13 | + 이름 : <input type="text" name="name" size="5"> | |
14 | + </label> | |
15 | + <label for="age"> | |
16 | + 나이 : <input type="text" name="age" size="2"> | |
17 | + </label> | |
18 | + <label for="tel"> | |
19 | + 연락처 : <input type="text" name="tel" size="8"> | |
20 | + </label><br> | |
21 | + <label for="area"> | |
22 | + 거주지 : | |
23 | + <select name="area"> | |
24 | + <option value="광주">광주</option> | |
25 | + <option value="서울">서울</option> | |
26 | + <option value="대전">대전</option> | |
27 | + <option value="전주">전주</option> | |
28 | + <option value="부산">부산</option> | |
29 | + <option value="울산">울산</option> | |
30 | + </select> | |
31 | + </label> | |
32 | + <label for="birth"> | |
33 | + 생년월일 : <input type="date" name="birth"> | |
34 | + </label> | |
35 | + <button type="submit">수정</button> | |
36 | + </form> | |
37 | +</body> | |
38 | +</html>(No newline at end of file) |
+++ 20220126/address_view.php
... | ... | @@ -0,0 +1,84 @@ |
1 | +<?php | |
2 | + require_once("Address.php"); | |
3 | + $address = new Address(); | |
4 | + $list = $address->getList(); | |
5 | + | |
6 | + $count = 0; | |
7 | +?> | |
8 | +<!doctype html> | |
9 | +<html lang="ko"> | |
10 | +<head> | |
11 | + <meta charset="UTF-8"> | |
12 | + <meta name="viewport" | |
13 | + content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> | |
14 | + <meta http-equiv="X-UA-Compatible" content="ie=edge"> | |
15 | + <title>주소록</title> | |
16 | + <style> | |
17 | + * {text-align: center; margin: 0 auto;} | |
18 | + #page {background-color: antiquewhite;} | |
19 | + th, td {padding: 10px;} | |
20 | + </style> | |
21 | +</head> | |
22 | +<body> | |
23 | + <div id="page"> | |
24 | + <h1>주소록 프로그램</h1> | |
25 | + <div> | |
26 | + <form action="address_insert.php" method="post"> | |
27 | + <label for="name"> | |
28 | + 이름 : <input type="text" name="name" size="5"> | |
29 | + </label> | |
30 | + <label for="age"> | |
31 | + 나이 : <input type="text" name="age" size="2"> | |
32 | + </label> | |
33 | + <label for="tel"> | |
34 | + 연락처 : <input type="text" name="tel" size="8"> | |
35 | + </label><br> | |
36 | + <label for="area"> | |
37 | + 거주지 : | |
38 | + <select name="area"> | |
39 | + <option value="광주">광주</option> | |
40 | + <option value="서울">서울</option> | |
41 | + <option value="대전">대전</option> | |
42 | + <option value="전주">전주</option> | |
43 | + <option value="부산">부산</option> | |
44 | + <option value="울산">울산</option> | |
45 | + </select> | |
46 | + </label> | |
47 | + <label for="birth"> | |
48 | + 생년월일 : <input type="date" name="birth"> | |
49 | + </label> | |
50 | + <button type="submit">추가</button> | |
51 | + </form> | |
52 | + </div> | |
53 | + <hr><br> | |
54 | + <table border="1"> | |
55 | + <tr> | |
56 | + <th>번호</th> | |
57 | + <th>이름</th> | |
58 | + <th>나이</th> | |
59 | + <th>연락처</th> | |
60 | + <th>거주지</th> | |
61 | + <th>생년월일</th> | |
62 | + <th>수정</th> | |
63 | + <th>삭제</th> | |
64 | + </tr> | |
65 | + <?php foreach ($list as $value) { | |
66 | +// $count = $count + 1; | |
67 | + ?> | |
68 | + <tr> | |
69 | + <td> <?php echo $value['no'] ?></td> | |
70 | + <td> <?php echo $value['name'] ?></td> | |
71 | + <td> <?php echo $value['age'] ?></td> | |
72 | + <td> <?php echo $value['tel'] ?></td> | |
73 | + <td> <?php echo $value['area'] ?></td> | |
74 | + <td> <?php echo $value['birth'] ?></td> | |
75 | + <td><a href="address_update_front.php?no=<?php echo $value['no'] ?>">수정</a></td> | |
76 | + <td><a href="address_delete.php?no=<?php echo $value['no'] ?>">삭제</a></td> | |
77 | + </tr> | |
78 | + <?php | |
79 | + } | |
80 | + ?> | |
81 | + </table> | |
82 | + </div> | |
83 | +</body> | |
84 | +</html>(No newline at end of file) |
--- 20220126/dbtest2.php
+++ 20220126/dbtest2.php
... | ... | @@ -18,27 +18,27 @@ |
18 | 18 |
} |
19 | 19 |
|
20 | 20 |
|
21 |
- echo "<br><hr>Update Test<br>"; |
|
22 |
- $result = $db->updateTest("김현식"); |
|
23 |
- if($result) { |
|
24 |
- echo "update Success!"; |
|
25 |
- }else { |
|
26 |
- echo "update Fail!"; |
|
27 |
- } |
|
21 |
+// echo "<br><hr>Update Test<br>"; |
|
22 |
+// $result = $db->updateTest("김현식", "안효원"); |
|
23 |
+// if($result) { |
|
24 |
+// echo "update Success!"; |
|
25 |
+// }else { |
|
26 |
+// echo "update Fail!"; |
|
27 |
+// } |
|
28 | 28 |
|
29 | 29 |
|
30 |
- echo "<br><hr>Delete Test<br>"; |
|
31 |
- $result = $db->deleteTest("안효원"); |
|
32 |
- if($result) { |
|
33 |
- echo "delete Success!"; |
|
34 |
- }else { |
|
35 |
- echo "delete Fail!"; |
|
36 |
- } |
|
37 |
- |
|
38 |
- |
|
39 |
- |
|
40 |
- |
|
41 |
- |
|
42 |
- |
|
43 |
- |
|
44 |
- |
|
30 |
+// echo "<br><hr>Delete Test<br>"; |
|
31 |
+// $result = $db->deleteTest("안효원"); |
|
32 |
+// if($result) { |
|
33 |
+// echo "delete Success!"; |
|
34 |
+// }else { |
|
35 |
+// echo "delete Fail!"; |
|
36 |
+// } |
|
37 |
+// |
|
38 |
+// |
|
39 |
+// |
|
40 |
+// |
|
41 |
+// |
|
42 |
+// |
|
43 |
+// |
|
44 |
+// |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?