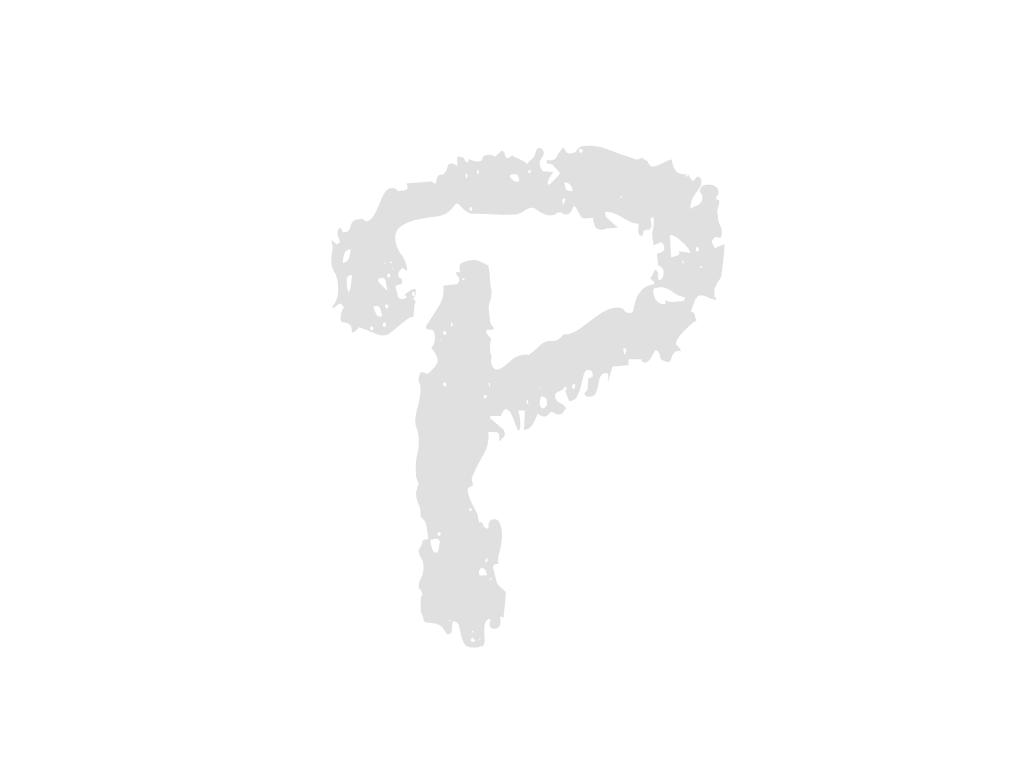
--- form_1.php
+++ form_1.php
... | ... | @@ -6,11 +6,21 @@ |
6 | 6 |
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> |
7 | 7 |
<meta http-equiv="X-UA-Compatible" content="ie=edge"> |
8 | 8 |
<title>Document</title> |
9 |
+ <style> |
|
10 |
+ #page {margin: 0 auto; background-color: antiquewhite; width: 720px;} |
|
11 |
+ h2 {text-align: center;} |
|
12 |
+ </style> |
|
9 | 13 |
</head> |
10 | 14 |
<body> |
11 |
- <form action="form_1_receive.php" method="post"> |
|
12 |
- 과일을 입력하세요 : <input type="text" name="fruits"> |
|
13 |
- <input type="submit"> |
|
14 |
- </form> |
|
15 |
+ <div id="page"> |
|
16 |
+ <h2>Data Send</h2> |
|
17 |
+ <hr> |
|
18 |
+ <form action="form_1_receive.php" method="get"> |
|
19 |
+ 과일을 입력하세요 : <input type="text" name="fruits"><br> |
|
20 |
+ 이름을 입력하세요 : <input type="text" name="name"><br> |
|
21 |
+ 생년월일을 입력하세요 : <input type="text" name="birth"><br> |
|
22 |
+ <input type="submit"> |
|
23 |
+ </form> |
|
24 |
+ </div> |
|
15 | 25 |
</body> |
16 | 26 |
</html>(No newline at end of file) |
--- form_1_receive.php
+++ form_1_receive.php
... | ... | @@ -1,4 +1,8 @@ |
1 | 1 |
<?php |
2 |
- $fruits = $_POST["fruits"]; |
|
2 |
+ $fruits = $_GET["fruits"]; |
|
3 |
+ $name = $_GET["name"]; |
|
4 |
+ $birth = $_GET["birth"]; |
|
3 | 5 |
|
4 |
- echo "과일 : " . $fruits;(No newline at end of file) |
|
6 |
+ echo "과일 : " . $fruits . "<br>"; |
|
7 |
+ echo "이름 : " . $name . "<br>"; |
|
8 |
+ echo "생년월일 : " . $birth . "<br>";(No newline at end of file) |
+++ form_2.php
... | ... | @@ -0,0 +1,46 @@ |
1 | +<!doctype html> | |
2 | +<html lang="kos"> | |
3 | +<head> | |
4 | + <meta charset="UTF-8"> | |
5 | + <meta name="viewport" | |
6 | + content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> | |
7 | + <meta http-equiv="X-UA-Compatible" content="ie=edge"> | |
8 | + <title>Document</title> | |
9 | + <style> | |
10 | + * {margin: 0 auto;} | |
11 | + #page {background-color: antiquewhite; width: 720px;} | |
12 | + table {margin-top: 100px;} | |
13 | + .send {text-align: center;} | |
14 | + .sendButton {width: 100%; height: 3em;} | |
15 | + </style> | |
16 | +</head> | |
17 | +<body> | |
18 | + <div id="page"> | |
19 | + <form action="form_2.receive.php" method="get"> | |
20 | + <table border="1"> | |
21 | + <tr> | |
22 | + <td><label for="userName">Name : </label></td> | |
23 | + <td><input type="text" name="userName" id="userName"></td> | |
24 | + </tr> | |
25 | + <tr> | |
26 | + <td><label for="userAge">Age : </label></td> | |
27 | + <td><input type="text" name="userAge" id="userAge"></td> | |
28 | + </tr> | |
29 | + <tr> | |
30 | + <td><label for="userArea">Area : </label></td> | |
31 | + <td> | |
32 | + <select name="userArea" id="userArea"> | |
33 | + <option value="seoul">seoul</option> | |
34 | + <option value="gwangju">gwangju</option> | |
35 | + <option value="busan">busan</option> | |
36 | + </select> | |
37 | + </td> | |
38 | + </tr> | |
39 | + <tr> | |
40 | + <td class="send" colspan="2"><input class="sendButton" type="submit" value="Send"></td> | |
41 | + </tr> | |
42 | + </table> | |
43 | + </form> | |
44 | + </div> | |
45 | +</body> | |
46 | +</html> |
+++ form_2.receive.php
... | ... | @@ -0,0 +1,10 @@ |
1 | +<?php | |
2 | + | |
3 | + $userName = $_GET["userName"]; | |
4 | + $userAge = $_GET["userAge"]; | |
5 | + $userArea = $_GET["userArea"]; | |
6 | + | |
7 | + echo "My name is <strong>" . $userName . "</strong><br>"; | |
8 | + echo "My Age is <strong>" . $userAge . "</strong><br>"; | |
9 | + echo "My Area is <strong>" . $userArea . "</strong><br>"; | |
10 | + |
+++ form_3.php
... | ... | @@ -0,0 +1,42 @@ |
1 | +<!doctype html> | |
2 | +<html lang=ko> | |
3 | +<head> | |
4 | + <meta charset="UTF-8"> | |
5 | + <meta name="viewport" | |
6 | + content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> | |
7 | + <meta http-equiv="X-UA-Compatible" content="ie=edge"> | |
8 | + <title>Document</title> | |
9 | + <style> | |
10 | + * {margin: 0 auto;} | |
11 | + #page {background-color: antiquewhite; width: 720px; text-align: center;} | |
12 | + .send {width: 28em;} | |
13 | + </style> | |
14 | +</head> | |
15 | +<body> | |
16 | + <div id="page"> | |
17 | + <form action="form_3.receive.php" method="post"> | |
18 | + Name : <input type="text" name="userName" placeholder="enter name here"><br> | |
19 | + Password : <input type="password" name="userPw"><br> | |
20 | + | |
21 | + <label><input type="radio" name="fruit" value="apple"> Apple</label> | |
22 | + <label><input type="radio" name="fruit" value="orange"> Orange</label> | |
23 | + <label><input type="radio" name="fruit" value="kiwi"> Kiwi</label> | |
24 | + <label><input type="radio" name="fruit" value="peach"> Peach</label><br> | |
25 | + | |
26 | + <label><input type="checkbox" name="fruits[]" value="apple"> Apple</label> | |
27 | + <label><input type="checkbox" name="fruits[]" value="orange"> Orange</label> | |
28 | + <label><input type="checkbox" name="fruits[]" value="kiwi"> Kiwi</label> | |
29 | + <label><input type="checkbox" name="fruits[]" value="peach"> Peach</label><br> | |
30 | + | |
31 | + <input type="date" name="date"><br> | |
32 | + | |
33 | + <textarea name="text" placeholder="Write Anything" cols="50" rows="10"></textarea><br> | |
34 | + | |
35 | + <input type="hidden" name="hiddenValue" value="InvisibleValue"> | |
36 | + | |
37 | + <!-- <input class="send" type="submit" value="Send"> --> | |
38 | + <button type="submit" class="send">Send</button> | |
39 | + </form> | |
40 | + </div> | |
41 | +</body> | |
42 | +</html>(No newline at end of file) |
+++ form_3.receive.php
... | ... | @@ -0,0 +1,37 @@ |
1 | +<?php | |
2 | + | |
3 | + $name = $_POST["userName"]; | |
4 | + $pw = $_POST["userPw"]; | |
5 | + $fruit = $_POST["fruit"]; | |
6 | + $fruits = $_POST["fruits"]; | |
7 | + $date = $_POST["date"]; | |
8 | + $text = $_POST["text"]; | |
9 | + $hiddenValue = $_POST["hiddenValue"]; | |
10 | + | |
11 | + echo "My name is <strong>" . $name . "</strong><br>"; | |
12 | + echo "Password is <strong>" . $pw . "</strong><br>"; | |
13 | + echo "Fruit is <strong>" . $fruit . "</strong><br>"; | |
14 | + | |
15 | + /*----------------------for-------------------------*/ | |
16 | + echo "<hr>"; | |
17 | + echo "<h3>for</h3>"; | |
18 | + $fruitsR = $_POST["fruits"]; | |
19 | + echo "Fruits is <strong>"; | |
20 | + for($i = 0; $i < count($fruitsR); $i++){ | |
21 | + echo $fruitsR[$i] . ", "; | |
22 | + } | |
23 | + echo "</strong><br>"; | |
24 | + /*-----------------------foreach------------------------*/ | |
25 | + echo "<hr>"; | |
26 | + echo "<h3>foreach</h3>"; | |
27 | + echo "Fruits is <strong>"; | |
28 | + foreach ($fruits as $i){ | |
29 | + print $i . ", "; | |
30 | + } | |
31 | + echo "</strong><br>"; | |
32 | + echo "<hr>"; | |
33 | + /*--------------------------------------------------*/ | |
34 | + | |
35 | + echo "Date is <strong>" . $date . "</strong><br>"; | |
36 | + echo "Text is <strong>" . $text . "</strong><br>"; | |
37 | + echo "HiddenValue is <strong>" . $hiddenValue . "</strong><br>"; |
+++ form_calc.php
... | ... | @@ -0,0 +1,31 @@ |
1 | +<!doctype html> | |
2 | +<html lang="ko"> | |
3 | +<head> | |
4 | + <meta charset="UTF-8"> | |
5 | + <meta name="viewport" | |
6 | + content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> | |
7 | + <meta http-equiv="X-UA-Compatible" content="ie=edge"> | |
8 | + <title>Document</title> | |
9 | + <style> | |
10 | + #page {margin: 0 auto; background-color: antiquewhite; width: 500px; text-align: center;} | |
11 | + #num1, #num2 {width: 30px;} | |
12 | + .calc {width: 100px; height: 50px;} | |
13 | + </style> | |
14 | +</head> | |
15 | +<body> | |
16 | + <div id="page"> | |
17 | + <h2>Calculator</h2> | |
18 | + <form action="form_calc_receive.php" method="post"> | |
19 | + <input type="text" name="num1" id="num1"> | |
20 | + <select name="op" id="op"> | |
21 | + <option value="+">+</option> | |
22 | + <option value="-">-</option> | |
23 | + <option value="*">X</option> | |
24 | + <option value="/">%</option> | |
25 | + </select> | |
26 | + <input type="text" name="num2" id="num2"><br><br> | |
27 | + <button class="calc" type="submit">Calc</button> | |
28 | + </form> | |
29 | + </div> | |
30 | +</body> | |
31 | +</html>(No newline at end of file) |
+++ form_calc_receive.php
... | ... | @@ -0,0 +1,39 @@ |
1 | +<!doctype html> | |
2 | +<html lang="ko"> | |
3 | +<head> | |
4 | + <meta charset="UTF-8"> | |
5 | + <meta name="viewport" | |
6 | + content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> | |
7 | + <meta http-equiv="X-UA-Compatible" content="ie=edge"> | |
8 | + <title>Document</title> | |
9 | + <style> | |
10 | + #page {margin: 0 auto; background-color: antiquewhite; width: 500px; text-align: center;} | |
11 | + .back {width: 100px; height: 50px;} | |
12 | + </style> | |
13 | +</head> | |
14 | +<body> | |
15 | + <div id="page"> | |
16 | + <h2>Calculating Result</h2><hr> | |
17 | + <?php | |
18 | + $num1 = $_POST["num1"]; | |
19 | + $op = $_POST["op"]; | |
20 | + $num2 = $_POST["num2"]; | |
21 | + | |
22 | + if ($num1 != null && $num2 != null){ | |
23 | + if($op == "+"){ | |
24 | + echo $num1 . "+" . $num2 . "=" . ($num1 + $num2); | |
25 | + }else if($op == "-"){ | |
26 | + echo $num1 . "-" . $num2 . "=" . ($num1 - $num2); | |
27 | + }else if($op == "*"){ | |
28 | + echo $num1 . "X" . $num2 . "=" . ($num1 * $num2); | |
29 | + }else{ | |
30 | + echo $num1 . "%" . $num2 . "=" . ($num1 / $num2); | |
31 | + } | |
32 | + }else{ | |
33 | + echo "Error!!!"; | |
34 | + } | |
35 | + ?> | |
36 | + <br><br><button class="back" onclick="history.back()">뒤로가기</button> | |
37 | + </div> | |
38 | +</body> | |
39 | +</html>(No newline at end of file) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?